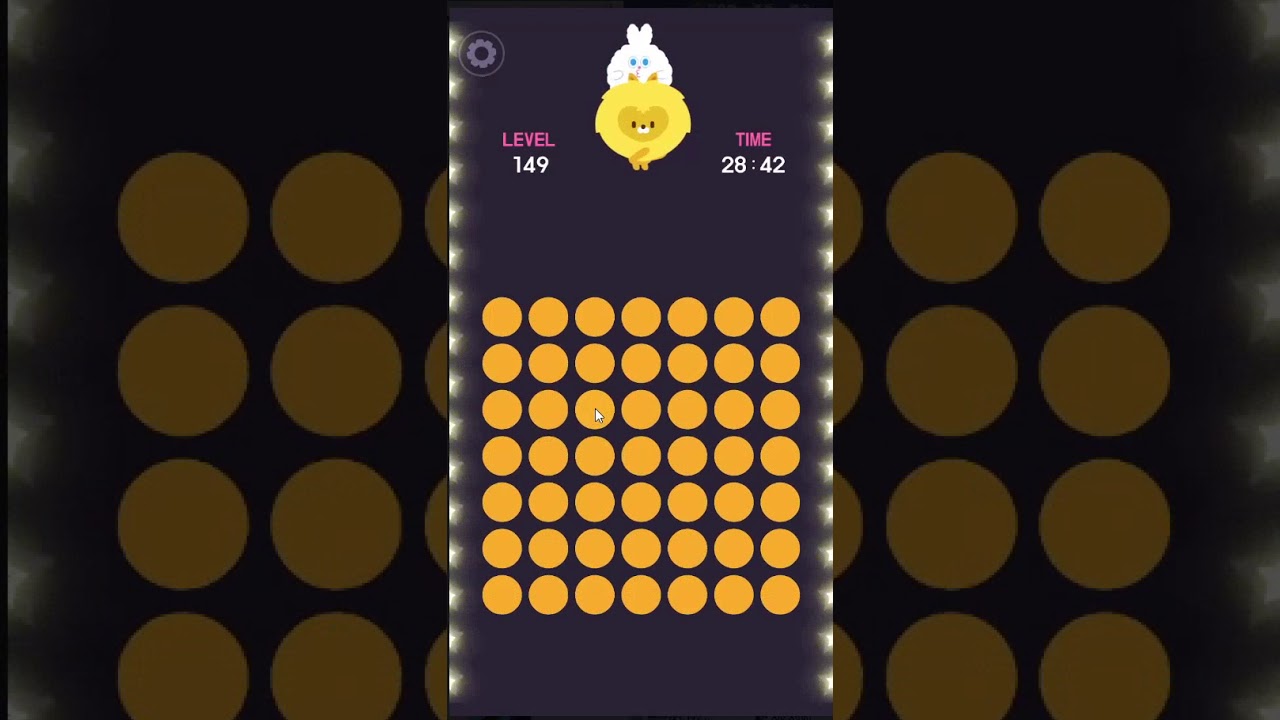
Lyto-Different-Color It’s basically a simple color recognition game, when I saw this game for the first time, I had the idea of using python and opencv to write this game assistant.
so first we need python, If you don’t know how to download python, you can go to this page
http://ouyangminwei.com/index.php/2021/09/13/how-to-download-python-in-windows/
We need opencv and pillow for image processing, numpy for array and mss for screenshot.
pip install numpy
pip install opencv-python
pip install pillow
pip install mss==2.0.22
Next, we need to get the game screen
# select screen range
mon = {'top': 179, 'left': 706, 'width': 1214-706, 'height': 1080-179}
sct = mss()
sct.get_pixels(mon)
while True:
# transform the screen information to numpy array
img = np.array(Image.frombytes('RGB', (sct.width, sct.height), sct.image))
# show the screen via opencv
cv2.imshow('test', np.array(img))
if cv2.waitKey(25) & 0xFF == ord('q'):
cv2.destroyAllWindows()
break
I want the program to find the ball in the screen first, and then get the color of the center of the ball, so that it can find the ball with a different color, there is a funcction in opencv, call HoughCircles, it can help us to find the circle in the image.
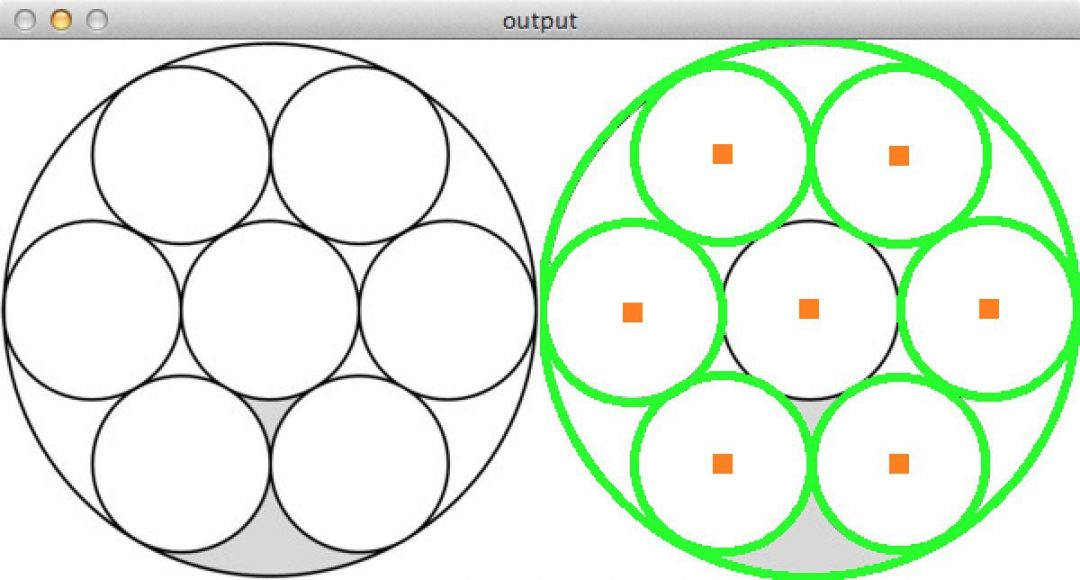
The usage is very simple, just throw the image into the function, well… and some parameters
# transform the image into gray scale to speed up the calculation
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# Houghcircles
circles = cv2.HoughCircles(gray, cv2.HOUGH_GRADIENT,1.2,10)
# circles will be something likes
# [(x1, y1, r1),(x1, y1, r1) ...]
# where x1 y1 is the position of circle's center and r is the radius
with circle’s center, we can get correspondging color
color = []
# loop every circle
for (x, y, r) in circles:
# store the circle's center color into 'colors'
colors.append(list(img[y,x,:]))
I write a function to find the different one, (there must be a better way of writing, but I only use a very dumb way)
def find_different(arr):
for num,i in enumerate(arr):
if (arr.count(i)==1):
return num
else:
return 0
At this point, we are done. All we need is to put them all together and draw the balls of different colors.
Let’s take a look at the results
Github : https://github.com/OuYangMinOa/Lyto-Different-Color